JMS Client
JMS Network Connections
Lookup a Connection Factory from JNDI
The purpose of connection factories is to hide physical connection properties from JMS clients. Instead, an object (a connection factory) is registered in JNDI from which physical connections are created.
Connection factories are created as a sub-entity of a JMS listener, so each connection factory is bound to a specific JMS listener and inherits the physical connection properties like port number and socket factory. Once a connection factory is created, it is registered automatically in JNDI under the specific name. There is always one default connection factory per JMS listener which is also registered in JNDI. The name of those connection factories consists of @, e.g. for a listener "plainsocket" on "router1", the connection factory is named "plainsocket@router1".
The following configuration of the JMS Swiftlet shows the definition of 1 listener and with some connection factories:
A connection factory contains various attribute definitions which define the behavior of the JMS clients, connecting via the connection factory:
Attribute | Default | Meaning |
---|---|---|
jms-client-id | null | Presets the JMS client id. If none is specified (default), a random client id is created. Note that it is not possible to create a durable subscriber without a client id or with a random one. The router will reject it. |
jms-default-delivery-mode | persistent | Presets the JMS default delivery mode for message producers. The default value is compliant with the JMS specification. |
jms-default-message-ttl | 0 | Presets the JMS default message time-to-live for message producers. The default value is compliant with the JMS specification. |
jms-default-message-priority | 4 | Presets the JMS default message priority for message producers. The default value is compliant with the JMS specification. |
jms-default-message-id-enabled | true | Presets whether a message id should be generated for produced messages. The default value is compliant with the JMS specification. |
jms-default-message-timestamp-enabled | true | Presets whether a time stamp should be generated for produced messages. The default value is compliant with the JMS specification. |
thread-context-classloader-for-getobject | false | Presets whether the thread contex class loader should be used during the deserialization of objects returned from an ObjectMessage' getObject() method. This is important if SwiftMQ is integrated into app servers which use different class loaders for message-driven beans. |
smqp-producer-reply-interval | 20 | Contains the interval after which a message producer waits on a reply and thus can act on flow control delays. This attributes takes only effect for non-persistent messages. The default value is optimal in conjunction with a smqp-consumer-cache-size of 500. |
smqp-consumer-cache-size | 500 | Contains the size of the client message cache of message consumers. The router sends up to this size without waiting for a reply. This attribute effects performance and the default value is optimal in conjunction with a smqp-producer-reply-interval of 20. |
smqp-consumer-cache-size-kb | 2048 | Limits the size of the cache per consumer to the specified value in KB. A value of -1 disables it. Limiting the size is useful to avoid out of memory errors if large messages are transfered. |
reconnect-enabled | depends | Enables transparent reconnect for JMS clients. Default "true" for SwiftMQ HA Router, "false" for non-HA Router. |
reconnect-max-retries | 50 | Specifies the max. retries for reconnect attemts. |
reconnect-delay | 10000 | Specifies the delay in ms between reconnect retries. |
duplicate-message-detection | depends | Enables/disables duplicate message detection at the client side. Must be enabled if reconnect is enabled. Default "true" for SwiftMQ HA Router, "false" for non-HA Router. |
duplicate-backlog-size | 30000 | Specifies the max. size of the duplicate backlog per connection (JMS Message Ids) at the client side. |
client-input-extend-size | 65536 | Specifies the extend size in bytes to increase the JMS client's network input buffer once it is full. |
client-output-buffer-size | 131072 | Specifies the initial size in bytes of the JMS client's network output buffer. |
client-output-extend-size | 65536 | Specifies the extend size in bytes to increase the JMS client's network output buffer once it is full. |
include java.util.*;
include javax.naming.*;
include javax.jms.*;
...
// Create the Hashtable with the JNDI environment properties
Hashtable env = new Hashtable();
env.put(Context.INITIAL_CONTEXT_FACTORY, "com.swiftmq.jndi.InitialContextFactoryImpl");
// Use a JMS listener on localhost:4001 for the JNDI connection
env.put(Context.PROVIDER_URL, "smqp://localhost:4001/timeout=10000");
InitialContext ctx = new InitialContext(env);
// Lookup a connection factory and a topic
TopicConnectionFactory tcf = (TopicConnectionFactory) ctx.lookup("TopicConnectionFactory");
Topic topic = (Topic) ctx.lookup("testtopic");
ctx.close();
// Create a topic connection
TopicConnection connection = tcf.createTopicConnection();
...
Creating a Connection Factory programmatically
It is also possible to create a JMS connection factory programmatically.
import com.swiftmq.jms.*;
...
Map props = new HashMap();
props.put(SwiftMQConnectionFactory.SOCKETFACTORY, "com.swiftmq.net.JSSESocketFactory");
props.put(SwiftMQConnectionFactory.HOSTNAME, "localhost");
props.put(SwiftMQConnectionFactory.PORT, "4001");
props.put(SwiftMQConnectionFactory.KEEPALIVEINTERVAL, "60000");
QueueConnectionFactory qcf = (QueueConnectionFactory) SwiftMQConnectionFactory.create(props);
TopicConnectionFactory tcf = (TopicConnectionFactory) SwiftMQConnectionFactory.create(props);
The Map
parameter of the create
method contains all necessary properties to create the required connection factory. The returned connection factory can be cast to both, Queue- and TopicConnectionFactory. The following table lists all possible properties which can be passed to the "create" method. All values have to be of String type, all property names are constants of SwiftMQConnectionFactory
.
Property | Default | Meaning |
---|---|---|
SwiftMQConnectionFactory. SOCKETFACTORY | - | Name of the Socket Factory class. |
SwiftMQConnectionFactory. HOSTNAME | - | Host Name. |
SwiftMQConnectionFactory. PORT | - | Port. |
SwiftMQConnectionFactory. RECONNECT_HOSTNAME2 | - | Host Name HA instance 2. |
SwiftMQConnectionFactory. RECONNECT_PORT2 | - | Port HA instance 2. |
SwiftMQConnectionFactory. KEEPALIVEINTERVAL | "0" | SMQP Keep Alive Interval |
SwiftMQConnectionFactory. CLIENTID | null | Presets the JMS client id. If none is specified (default), a random client id is created. Note that it is not possible to create a durable subscriber without a client id or with a random one. The router will reject it. |
SwiftMQConnectionFactory. SMQP_PRODUCER_REPLY_INTERVAL | "20" | Contains the interval after which a message producer waits on a reply and thus can act on flow control delays. This attributes takes only effect for non-persistent messages. The default value is optimal in conjunction with a smqp-consumer-cache-size of 500. |
SwiftMQConnectionFactory. SMQP_CONSUMER_CACHE_SIZE | "500" | Contains the size of the client message cache of message consumers. The router sends up to this size without waiting for a reply. This attribute effects performance and the default value is optimal in conjunction with a smqp-producer-reply-interval of 20. |
SwiftMQConnectionFactory. SMQP_CONSUMER_CACHE_SIZE_KB | "2048" | Limits the size of the cache per consumer to the specified value in KB. A value of -1 disables it. Limiting the size is useful to avoid out of memory errors if large messages are transfered. |
SwiftMQConnectionFactory. JMS_DELIVERY_MODE | String.valueOf(Message. DEFAULT_DELIVERY_MODE) | Presets the JMS default delivery mode for message producers. The default value is compliant with the JMS specification. |
SwiftMQConnectionFactory. JMS_PRIORITY | String.valueOf(Message. DEFAULT_PRIORITY) | Presets the JMS default message priority for message producers. The default value is compliant with the JMS specification. |
SwiftMQConnectionFactory. JMS_TTL | String.valueOf(Message. DEFAULT_TIME_TO_LIVE) | Presets the JMS default message time-to-live for message producers. The default value is compliant with the JMS specification. |
SwiftMQConnectionFactory. JMS_MESSAGEID_ENABLED | "true" | Presets whether a message id should be generated for produced messages. The default value is compliant with the JMS specification. |
SwiftMQConnectionFactory. JMS_TIMESTAMP_ENABLED | "true" | Presets whether a time stamp should be generated for produced messages. The default value is compliant with the JMS specification. |
SwiftMQConnectionFactory. USE_THREAD_CONTEXT_CLASSLOADER | "false" | Presets whether the thread contex class loader should be used during the deserialization of objects returned from an ObjectMessage' getObject() method. This is important if SwiftMQ is integrated into app servers which use different class loaders for message-driven beans. |
SwiftMQConnectionFactory. RECONNECT_ENABLED | "false" | Enables transparent reconnect for JMS clients. |
SwiftMQConnectionFactory. RECONNECT_MAX_RETRIES | 10 | Specifies the max. retries for reconnect attemts. |
SwiftMQConnectionFactory. RECONNECT_RETRY_DELAY | 10000 | Specifies the delay in ms between reconnect retries. |
SwiftMQConnectionFactory. DUPLICATE_DETECTION_ENABLED | "false" | Enables/disables duplicate message detection at the client side. Must be enabled if reconnect is enabled. |
SwiftMQConnectionFactory. DUPLICATE_BACKLOG_SIZE | 30000 | Specifies the max. size of the duplicate backlog per connection (JMS Message Ids) at the client side. |
SwiftMQConnectionFactory. INPUT_BUFFER_SIZE | "131072" | Specifies the initial size in bytes of the JMS client's network input buffer. |
SwiftMQConnectionFactory. INPUT_EXTEND_SIZE | "65536" | Specifies the extend size in bytes to increase the JMS client's network input buffer once it is full. |
SwiftMQConnectionFactory. OUTPUT_BUFFER_SIZE | "131072" | Specifies the initial size in bytes of the JMS client's network output buffer. |
SwiftMQConnectionFactory. OUTPUT_EXTEND_SIZE | "65536" | Specifies the extend size in bytes to increase the JMS client's network output buffer once it is full. |
Transparent Reconnect
Overview
If a SwiftMQ Router is shut down (e.g. due to an upgrade) and restarted or a network problem occurred, JMS clients can transparent reconnect to the same SwiftMQ Router without any additional coding.
SwiftMQ Router provides transparent reconnect to JMS clients to the same SwiftMQ Router, including recovery of all transactions in transit (incl. XA transactions). Processing continues where it stops before the reconnect. Persistent messages are never lost or delivered twice if the router was orderly shut down or uses disk-sync for its transaction log (see Store Swiftlet). The message sequence is guaranteed.
Non-persistent messages in transit may be lost during reconnecting.
Setting the Delivery Mode / Avoid using Queue Persistency Overwrite Mode
To send persistent messages, you must always use the default delivery mode (persistent according to the JMS specification) or specify it explicitly as a parameter of the send/publish method. This guarantees that the send/publish method is processed synchronously and returns only when the router saved the message to disk. Do not send/publish with a default delivery mode of non-persistent and use the Queue Persistency Overwrite Mode to set the message as persistent. In that case the delivery is performed asynchronously and the send/publish method returns before the message has been saved to disk and can lead to message lost.
The behavior of receive(timeout)
during Reconnect
A call to receive(timeout)
may time out during reconnect and return null if the specified timeout is less than the reconnect time.
Duplicate Message Detection and JMS Message Id
Duplicate message detection uses the JMS Message-Id. Since it is allowed in JMS to disable the JMS Message-Id, it must be enabled (which is the default) when using transparent reconnect. Otherwise, duplicate message detection will not work.
Duplicate Message Detection on Queues/Queue Controller
Duplicate message detection for all queues and queue controllers is enabled by default.
Duplicate Message Detection on Connection Factories
Duplicate message detection for JMS connection factories are disabled at a SwiftMQ Router (non-HA). It must be enabled for transparent reconnect.
Request/Reply with temporary Destinations
Temporary destinations (temp. queues, temp. topics) are reconstructed during reconnect. They are still valid but now bound to different physical temp. queues at the new router instance. Since non-persistent messages are lost, a consumer may not receive the expected reply. Further, the consumer of this destination must inform the producer about the new temp. queue or topic after a reconnect, e.g. by sending the same temporary queue or topic object as JMSReplyTo
for the next request.
To avoid that, regular queues (PtP) or durable subscribers (Pub/Sub) with persistent messages can be used for request/reply.
Reconnect Configuration
The reconnect configuration is specified in the connection factory. It contains additional attributes for reconnecting and retrying. These attributes are disabled by default. The pictures marks are also attributed to a 2nd HA instance. These attributes are for SwiftMQ HA Router only. Transparent reconnect only requires attributes "Reconnect Enabled", "Reconnect Delay", "Reconnect Max. Retries", and "Duplicate Message Detection" of the connection factory.
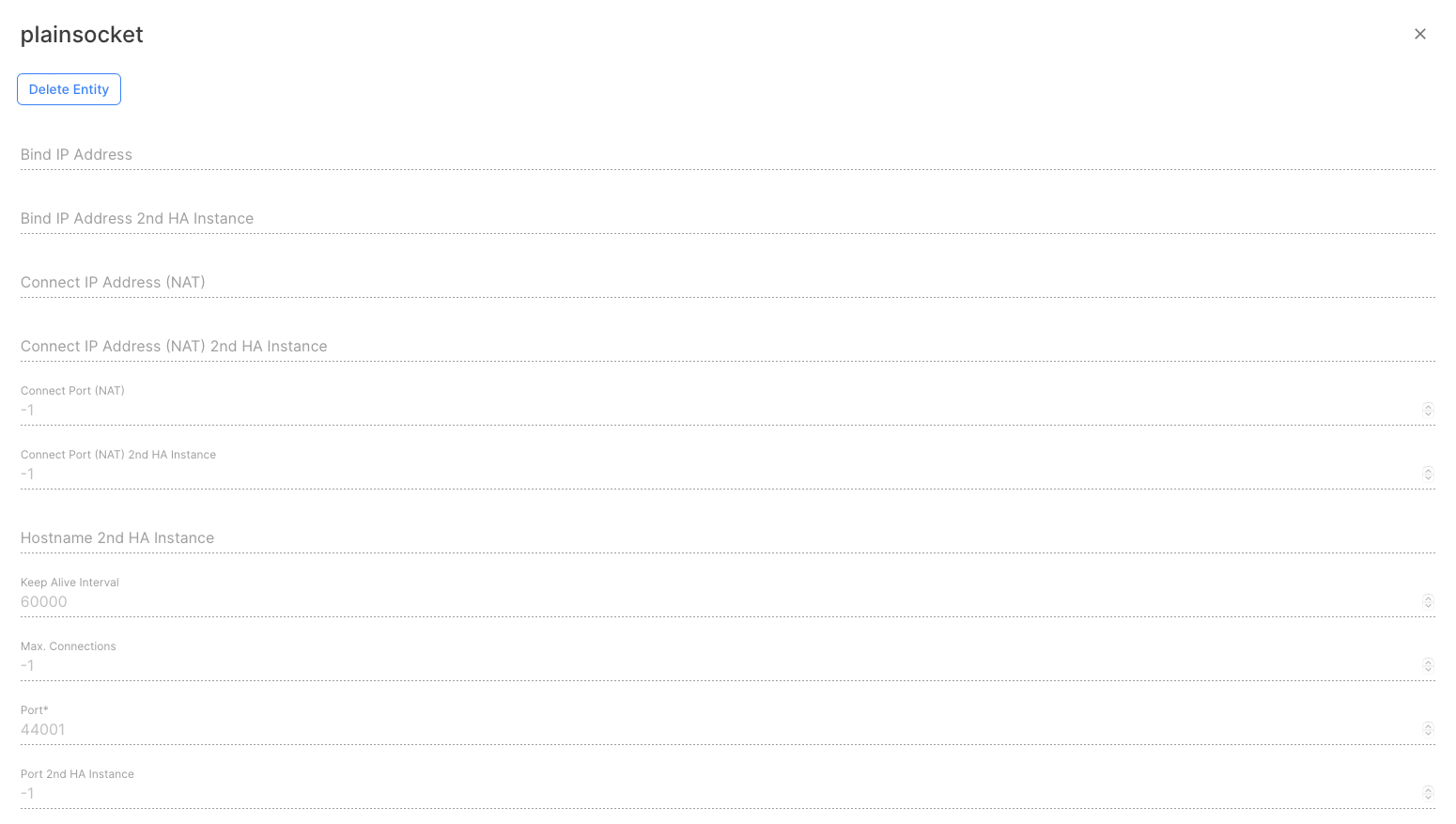
JMS listener “plainsocket” [SwiftMQ Explorer App, Flow Director]

Reconnect attributes connection factory [SwiftMQ Explorer App, Flow Director]
The reconnect attributes of a connection factory are read-only. To change it via SwiftMQ Explorer/CLI, the connection factory must be deleted and recreated (and the configuration must be saved).
Using a Reconnect Listener
To receive a reconnect event after a reconnect has been completed, a JMS client can register a reconnect listener at a SwiftMQ connection. The interface is:
package com.swiftmq.jms;
public interface ReconnectListener
{
public void reconnected(String host, int port);
}
"host" and "port" are the host and port of the new router instance after the reconnect.
Example to register a reconnect listener:
javax.jms.Connection connection = ...
((com.swiftmq.jms.SwiftMQConnection) connection).addReconnectListener(new ReconnectListener()
{
public void reconnected(String host, int port)
{
// Do stuff
}
});
Reconnect Debug Output
To see debug output during connect and reconnect, set system property
-Dswiftmq.reconnect.debug=true
at your client's JVM command line. Debug output goes to System.out.
JMS IntraVM Connections
Introduction
The intra-VM JMS client is a client which is connected with a SwiftMQ router via an intra-VM connection. This is not a socket connection but a virtual connection from the JMS client part to an intra-VM connector in the Network Swiftlet. Both the SwiftMQ router and one or more JMS clients are running in the same virtual machine. This requires to start the router as the first action to be able to start intra-VM clients thereafter.
The actual application code of an intra-VM JMS client doesn't differ from a remote JMS client, except the JNDI provider URL and the name of the connection factory to use:
include java.util.*;
include javax.naming.*;
include javax.jms.*;
...
// Create the Hashtable with the JNDI environment properties
Hashtable env = new Hashtable();
env.put(Context.INITIAL_CONTEXT_FACTORY, "com.swiftmq.jndi.InitialContextFactoryImpl");
// Use an intraVM JMS connection for the JNDI connection
env.put(Context.PROVIDER_URL, "smqp://intravm/timeout=10000");
InitialContext ctx = new InitialContext(env);
// Lookup a connection factory and a topic
TopicConnectionFactory tcf = (TopicConnectionFactory) ctx.lookup("IVMTopicConnectionFactory");
Topic topic = (Topic) ctx.lookup("testtopic");
ctx.close();
// Create a topic connection
TopicConnection connection = tcf.createTopicConnection();
...
The JNDI provider URL here is smqp://intravm/timeout=10000
where intravm
is a keyword and instructs SwiftMQ's JNDI context to create an intra-VM connection. The IVMTopicConnectionFactory
is defined as an intra-VM connection factory. Once the application creates a connection from it, it creates an intra-VM connection.
Launching SwiftMQ
Before an intra-VM JMS client can be used, the SwiftMQ router must be started. For example, a private method with the startup statements can be used and can be called during the initialization.
Example:
import com.swiftmq.swiftlet.*;
...
private static void startSwiftMQ(String workingDir, String configFile, boolean hook)
throws Exception
{
if (!hook)
System.setProperty("swiftmq.shutdown.hook","false");
SwiftletManager.getInstance().setWorkingDirectory(workingDir);
SwiftletManager.getInstance().startRouter(configFile);
}
private static void shutdownSwiftMQ()
{
SwiftletManager.getInstance().shutdown();
}
public static void main(String[] args)
{
// Start SwiftMQ
try {
startSwiftMQ("/opt/swiftmq/scripts",
"../config/routerconfig.xml",
true);
} catch (Exception e)
{
e.printStackTrace();
System.exit(-1);
}
// Start my application
...
// Shutdown SwiftMQ
shutdownSwiftMQ();
}
Shutdown Hook
A shutdown hook must not be registered if the application registers its own shutdown hook and callsSwiftletManager.getInstance().shutdown()
within that hook.
Connection Factories
Connection factories for intra-VM connections are located below the element <intravm-connection-factories>
in the JMS Swiftlet configuration. They are similar to normal connection factories, except they have no network buffer attributes.
The following configuration of the JMS Swiftlet shows the definition of 2 intra-VM connection factories:
<swiftlet name="sys$jms">
<intravm-connection-factories>
<intravm-connection-factory name="IVMQueueConnectionFactory"/>
<intravm-connection-factory name="IVMTopicConnectionFactory"/>
</intravm-connection-factories>
<listeners>
<listener name="plainsocket" port="4001">
<connection-factories>
<connection-factory name="QueueConnectionFactory"/>
<connection-factory name="TopicConnectionFactory"/>
<connection-factory name="plainsocket@router1"/>
</connection-factories>
<host-access-list/>
</listener>
</listeners>
</swiftlet>
Client-Side Threadpools
An intra-VM JMS client utilizes 2 thread pools, one to drive sessions, and one to drive connection outbound writes (client to router). In contrast to a remote JMS client, threads of an intra-VM JMS client are scheduled in thread pools from the Threadpool Swiftlet.
The following thread pools are used from intra-VM JMS clients:
jms.ivm.client.connection
jms.ivm.client.session
The default configuration of these pools are:
<pool name="jms.ivm.client.connection" kernel-pool="true" max-threads="10" min-threads="1">
<threads>
<thread name="sys$jms.client.connection.%"/>
</threads>
</pool>
<pool name="jms.ivm.client.session" kernel-pool="true" max-threads="10" min-threads="1">
<threads>
<thread name="sys$jms.client.session.%"/>
</threads>
</pool>
Details of that configuration are described in the Threadpool Swiftlet documentation.
JMS Pooling for Spring's JmsTemplate and plain JMS
Introduction
The Spring Framework provides a JMS abstraction called JmsTemplate
. Unfortunately, this class acts against a JMS provider in the same way as it acts against JMS in an application server. For example, to send a message, a new connection, session, and producer is created, the message is sent and everything is closed. This is done for every single message.
This behavior is necessary for a managed EJB (J2EE) environment, however, a J2EE application server pools its resources under the covers but if JmsTemplate
is used against a plain JMS provider, it turns inefficient and slow.
Spring itself provides a class called SingleConnectionFactory
which uses a single shared JMS connection but this does not avoid the creation of sessions, producers, and consumers.
SwiftMQ provides a SingleSharedConnectionFactory
which uses a shared JMS connection and pools JMS sessions, producer, and consumer objects.
Using it with Spring
To use SingleSharedConnectionFactory
with Spring, add it to your client's classpath and use classcom.swiftmq.jms.springsupport.SingleSharedConnectionFactory
as your connection factory as shown in this example:
<bean id="jmsConnectionFactory"
class="org.springframework.jndi.JndiObjectFactoryBean"
lazy-init="true">
<property name="jndiTemplate">
<ref bean="jndiTemplate"/>
</property>
<property name="jndiName">
<value>plainsocket@router1</value>
</property>
</bean>
<bean id="testqueue"
class="org.springframework.jndi.JndiObjectFactoryBean">
<property name="jndiTemplate">
<ref bean="jndiTemplate"/>
</property>
<property name="jndiName">
<value>testqueue@router1</value>
</property>
</bean>
<bean id ="singleSharedConnectionFactory"
class="com.swiftmq.jms.springsupport.SingleSharedConnectionFactory"
destroy-method="destroy" >
<property name="targetConnectionFactory" ref="jmsConnectionFactory"/>
<property name="poolExpiration" value="120000"/>
<property name="clientId" value="test"/>
</bean>
<bean id="jmsTemplate"
class="org.springframework.jms.core.JmsTemplate">
<property name="connectionFactory">
<ref bean="singleSharedConnectionFactory"/>
</property>
<property name="defaultDestination">
<ref bean="testqueue"/>
</property>
</bean>
<bean id="springSender"
class="SpringSender">
<property name="jmsTemplate">
<ref bean="jmsTemplate"/>
</property>
</bean>
SingleSharedConnectionFactory
uses a single shared JMS connection. This single connection provides pooling for JMS sessions where each JMS session pools producers and consumers.
The class provides 3 properties. One is targetConnectionFactory
which needs to be set to a "real" connection factory obtained from SwiftMQ's JNDI. It is used to create the single shared JMS connection. The next is poolExpiration
which defines the time in milliseconds after which a pooled object (session, producer, consumer) will expire when it has not being used within this time. So the pools grows and shrinks dependent on the load. Default for poolExpiration
is 60000 ms (1 minute). The last is clientId
which is optional and can be set to the JMS client id.
To free all resources held from SingleSharedConnectionFactory
define the destroy
-method:
<bean id ="singleSharedConnectionFactory"
class="com.swiftmq.jms.springsupport.SingleSharedConnectionFactory"
destroy-method="destroy" >
<property name="targetConnectionFactory" ref="jmsConnectionFactory"/>
<property name="poolExpiration" value="120000"/>
<property name="clientId" value="test"/>
</bean>
Using it with plain JMS
SingleSharedConnectionFactory
can also be used from plain JMS clients (without Spring). Just wrap your connection factory with SingleSharedConnectionFactory
and you have pooling:
Hashtable env = new Hashtable();
env.put(Context.INITIAL_CONTEXT_FACTORY,"com.swiftmq.jndi.InitialContextFactoryImpl");
env.put(Context.PROVIDER_URL,smqpURL);
InitialContext ctx = new InitialContext(env);
QueueConnectionFactory connectionFactory = new SingleSharedConnectionFactory((QueueConnectionFactory)ctx.lookup(qcfName));
SingleSharedConnectionFactory
implements ConnectionFactory
, QueueConnectionFactory
, TopicConnectionFactory
and can be used for all JMS messaging domains.
To free all resources held from SingleSharedConnectionFactory
call destroy
:
((SingleSharedConnectionFactory)connectionFactory).destroy();
Debugging
Debugging of the library can be enabled by setting this System Property to true:
-Dswiftmq.springsupport.debug=true
Debug output goes to System.out
.
List of SwiftMQ Exceptions
The following table provides a list of all exceptions which might be delivered to a JMS client. All exceptions extend javax.jms.JMSException
.
Exception | Meaning |
---|---|
com.swiftmq.jms.ConnectionLostException | The connection to the router was disconnected. This exception will also be delivered to a registered javax.jms.ExceptionListener. |
com.swiftmq.jms.RequestTimeoutException | A SMQP request timed out, a reply wasn't received within the time configured by system property "swiftmq.request.timeout". Can be thrown from any method that interacts with the router. |
com.swiftmq.swiftlet.auth.AuthenticationException | There was a problem with authentication, e.g. user unknow, password wrong. Since this exception extends javax.jms.JMSSecurityException, it is usually not necessary to catch it. Thrown from the connection factory's methods to create a connection. |
com.swiftmq.swiftlet.auth.ResourceLimitException | The client tried to exceed a predefined resource limit (assigned resource limit group of the particular user). Thrown from the relevant connection/session methods to create producer, consumer, temporary queues/topics. |
com.swiftmq.swiftlet.queue.QueueLimitException | The maximum number of messages defined for this queue has been reached. Thrown from send or commit methods of a message producer. |
com.swiftmq.swiftlet.queue.QueueException | A generic queue exception. Should only be thrown on serious issues such as disk full etc. |
Miscellaneous client-side Options
Adjusting Network Buffers
SwiftMQ manages its own network buffers on both sides of the wire (client and router). The length of the data sent to the network is determined by the underlying SMQP protocol handlers. Only complete requests are sent. Those requests can also be a bulk request which contains collected single requests. Before a request is sent, it is written to a network buffer. The receiving side receives the data into a network buffer as well. These buffers are adjusted automatically. However, the adjustment may take time when transferring large messages, so it might be necessary to configure buffer/extend sizes that meet the requirements more specifically.
Network buffers are initially created with their initial size which is 128 KB by default. If the protocol handler detects that the buffer is full before it can flush it, it will extend the buffer and continues writing until the request is finished or the buffer fills up again which then leads to another extend. The default extend size is 64 KB.
The initial buffer size and the extend size can be specified for:
Router input
Router output
Client input
Client output
The input/output sizes for the router is specified in the JMS listener definition. The input/output sizes for the client are specified in the connection factory definition. So it's possible to adjust network buffers for both sides by creating the appropriate JMS listeners and connection factories.
All network buffers are bound to a connection so each connection has one input and one output buffer. If there is a high number of concurrent connections, the overall memory consumption for the network buffers will go up.
Network output buffers (router output, client output) are bound via a ThreadLocal
to the thread which writes to the output stream. On the router side, these are threads from pool jms.connection
and on the client-side it is the connection
thread pool (see below, "Client-Side Threadpools"). The number of network output buffers is therefore the number of threads.
SMQP Protocol Version
It is possible to specify a particular SMQP protocol version to connect to a SwiftMQ Router. This is only necessary if it is required to connect with a higher versioned JMS client (e.g. CLI) to an older SwiftMQ Router, e.g. if the SwiftMQ Router can't be upgraded due to some reasons. To use the most recent client-side features, the SMQP protocol version can be set with the following system property:
-Dswiftmq.smqp.version=<version>
where "version" is one of the following:
400
500
510
600
610
630
750
Version 750 is the latest version and default.
Client-Side Threadpools
A JMS client utilizes 3 thread pools, one to drive sessions, one to drive connection outbound writes (client to router), and one to handle transparent reconnects. All pools are similar to the pools used from the Threadpool Swiftlet (they have the same attributes), however, the pool configuration takes place by system properties, to be defined on the client's command line. The default settings are optimal, so a change would be seldom.
Session Threadpool
System Property | Default | Meaning |
---|---|---|
swiftmq.pool.session.threads.min | 5 | Minimum threads. This number is prestarted. |
swiftmq.pool.session.threads.max | 50 | Maximum threads. Default changed to 50 starting from release 7.6.0. |
swiftmq.pool.session.priority | Thread.NORM_PRIORITY | Thread Priority. |
swiftmq.pool.session.queue.length | 1 | Pool length (back log), after which an additional thread is started. |
swiftmq.pool.session.threads.add | 1 | The number of additional threads to start if the queue length is reached. |
swiftmq.pool.session.idle.timeout | 120000 | Idle timeout after which an additional thread will die. |
Connection Threadpool
System Property | Default | Meaning |
---|---|---|
swiftmq.pool.connection.threads.min | 5 | Minimum threads. This number is prestarted. |
swiftmq.pool.connection.threads.max | 50 | Maximum threads. Default changed to 50 starting from release 7.6.0. |
swiftmq.pool.connection.priority | Thread.NORM_PRIORITY | Thread Priority. |
swiftmq.pool.connection.queue.length | 1 | Pool length (back log), after which an additional thread is started. |
swiftmq.pool.connection.threads.add | 1 | The number of additional threads to start if the queue length is reached. |
swiftmq.pool.connection.idle.timeout | 120000 | Idle timeout after which an additional thread will die. |
Connector Threadpool
System Property | Default | Meaning |
---|---|---|
swiftmq.pool.connector.threads.min | 1 | Minimum threads. This number is prestarted. |
swiftmq.pool.connector.threads.max | 10 | Maximum threads. |
swiftmq.pool.connector.priority | Thread.NORM_PRIORITY | Thread Priority. |
swiftmq.pool.connector.queue.length | 1 | Pool length (back log), after which an additional thread is started. |
swiftmq.pool.connector.threads.add | 1 | The number of additional threads to start if the queue length is reached. |
swiftmq.pool.connector.idle.timeout | 120000 | Idle timeout after which an additional thread will die. |